How to make automatic updates work with Tauri v2 and GitHub
Tauri is a great framework for building desktop applications with web technologies, backed by Rust. An updater comes packed with Tauri and is pretty straightforward to use. The Tauri v1 docs cover it quite nicely. But there are some changes in Tauri v2 that might make it a bit confusing to set up the updater. Here’s how I made it work for my Tauri v2 app using GitHub.
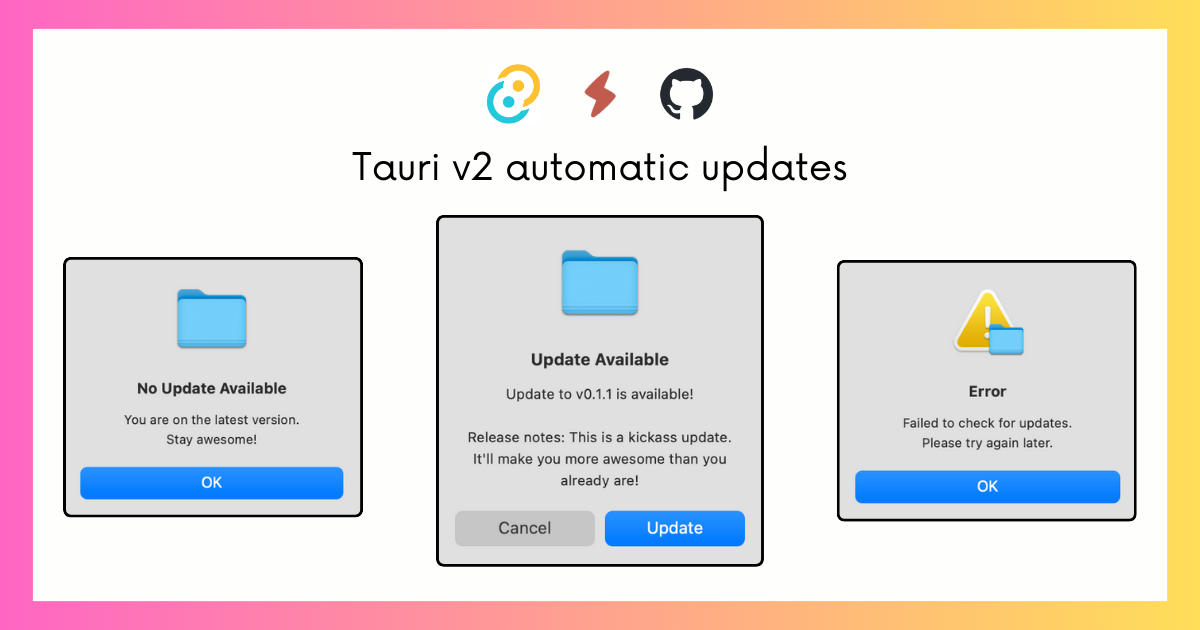
It just works.
TL;DR
The developer side is a manual process:
- You build the binaries locally.
- Then create a release on GitHub and upload the binaries.
- Then update the
latest.json
file in your GitHub repository or GitHub gist with the new version, release notes, signature and download URL.
Alternatively, you can use GitHub Actions to build the binaries and update the latest.json
file automatically. More on that later.
The user side is fully automatic:
- When the app starts, it will ping the
latest.json
file to check for updates, and show a dialog box if an update is available. Or the user can click on the “Check for Updates” button to manually check for updates. - The app will download the new binaries, verify the signature, and replace the old binaries with the new ones. It will then restart to apply the update.
1. The Groundwork
The layout:
- Local: Insert the updater code into your app’s source code. Then build the distributable binaries on your machine.
- GitHub: Publish the binaries on GitHub in a release in the project’s repository (or a separate public repository, if the project is private). Update the publicly accessible
latest.json
file with the new version details. - User: The app will ping the
latest.json
file to check for updates and show a dialog box if an update is available. It will then auto-install the update and restart the app.
Things to do:
- Make sure you have a working Tauri v2 project. Follow the official docs to see how.
- Set the same project version in the
Cargo.toml
,tauri.conf.json
andpackage.json
files. - Make sure you have a GitHub repository for your project.
- If your project repo is private, create a new public repository where you will publish your releases.
2. Add Updater to your Project
- Add the updater plugin to your project.
|
|
- Install the
npm
packages.
|
|
- Create private and public keys for the updater. Store the private key and the password in a secure place, maybe even a password manager like Bitwarden. Remember the path to the private key file and the password for the private key. You will need them for Step 7.
|
|
- Add the code for the updater to your front-end. You can do it however you like. Most simply:
- Add a
checkForAppUpdates
function in your app. - Add a “check for updates” button somewhere in your app that calls the above function. Also, automatically call this function when the app loads.
- This function will check for an update and then show a dialog box if an update is available. The user can then choose to update or not.
- If the user clicked on the check for updates button, show another dialog box if the update is not available. (Make sure to pass
true
to thecheckForAppUpdates
function below in this case.)
|
|
- Make sure to add the relevant permissions in your
capabilities > main.json
file.
|
|
- In your
tauri.conf.json
, add the updater configuration. Make sure to add the raw URL to the endpoints array in the config.
|
|
- Add the required environment variables to your shell before you build the binaries:
TAURI_SIGNING_PRIVATE_KEY
- The path to the private key file or the private key itself.TAURI_SIGNING_PRIVATE_KEY_PASSWORD
- The password for the private key.
Here is how you do it:
|
|
3. Set up GitHub
First, choose whether you are going to place the latest.json
file in your project repository or in a GitHub Gist.
The latest.json
file should look something like this:
|
|
version
can be with or without the leadingv
.notes
can be any string.pub_date
should be in the formatYYYY-MM-DDTHH:MM:SSZ
.platforms
can contain the platforms you are targeting. The keys should be the platform names and the values should be objects withsignature
andurl
keys.signature
should be the content of the signature file generated when you build for the respective platform.url
should be the URL to the binary file from the release. Don’t accidentally put the URL of the release page itself.
Test The Flow!
- Set the project version to
0.0.1
inCargo.toml
,tauri.conf.json
andpackage.json
. - Set the
TAURI_SIGNING_PRIVATE_KEY_PASSWORD
andTAURI_SIGNING_PRIVATE_KEY
environment variables in your shell. - Build the binaries.
- Create a release on GitHub with the binaries.
- Update the
latest.json
file in your GitHub repository or GitHub Gist with the new version, release notes, signature and download URL.
Now, repeat the above steps with a new version, say 0.0.2
.
At this point, your GitHub repo will have two releases, and the latest.json
file will have the details of the latest release.
Moment of Truth
- Download the app from the first release (
0.0.1
). - Run the app. Click on About and check the version. It should be
0.0.1
. - The app will check for updates and show a dialog box if an update is available. If it doesn’t, click on the “Check for Updates” button.
- Click the Update button. The app will download the update in the background and restart when done.
- The app will now be updated to the latest version (
0.0.2
). Verify by clicking on About.
Congratulations! You have successfully set up the auto-updater for your Tauri v2 app.
Appendix: Automate the Process
You can automate the process of building the binaries and updating the latest.json
file using GitHub Actions. This way, you don’t have to manually build the binaries and update the latest.json
file every time you release a new version.
I haven’t yet set it up for myself. When I do, I’ll update this post with the steps.
Questions?
If you have any questions related to Tauri, or need help with setting up the auto-updater for your Tauri v2 app, get in on the Tauri Discord server and ask away. The community is very helpful and responsive.